Managing data from telematics devices can be challenging—especially when every GPS tracker or sensor speaks a different “language.” With hundreds of device manufacturers using proprietary protocols, building and maintaining a unified telematics ecosystem becomes exponentially complex with each new device type.
Today, we're introducing the Navixy Generic Protocol (NGP)—our answer to the fragmentation challenge in the telematics industry. We’ll explore how the Navixy Generic Protocol transforms telematics, how it works, and the ways it can benefit your operations.
The integration problem: why standard protocols matter
Anyone who has built telematics systems knows the pain: each new device integration requires custom protocol parsing, specific data mapping, and dedicated maintenance. This creates three fundamental challenges:
Engineering bottleneck | Each manufacturer implements their own protocol, optimized for their specific use case. This means development teams spend more time writing and maintaining parsers than building valuable features. As your device portfolio grows, so does the engineering effort required just to keep the system running. |
Data consistency problem | Different protocols represent similar data in wildly different ways. What one device calls "speed" might be "velocity" in another, stored in different units and formats. This inconsistency makes it nearly impossible to build reliable analytics and reporting systems without complex transformation layers. |
Scale and maintenance tax | Every new device type you add to your system comes with its own protocol quirks and edge cases. This multiplication of complexity doesn't just slow down growth—it actively works against it, as each new integration increases the maintenance burden on your entire system. |
These challenges demanded a fresh approach: a protocol that could serve as a universal language for telematics while remaining flexible enough to accommodate device-specific capabilities. That's exactly what we set out to build with NGP.
How NGP bridges the telematics data gap
NGP serves as a universal translator for the IoT ecosystem, handling data flow throughout the entire processing pipeline. At its core, NGP standardizes two critical stages of data handling: upstream data collection from devices and downstream distribution of processed data.
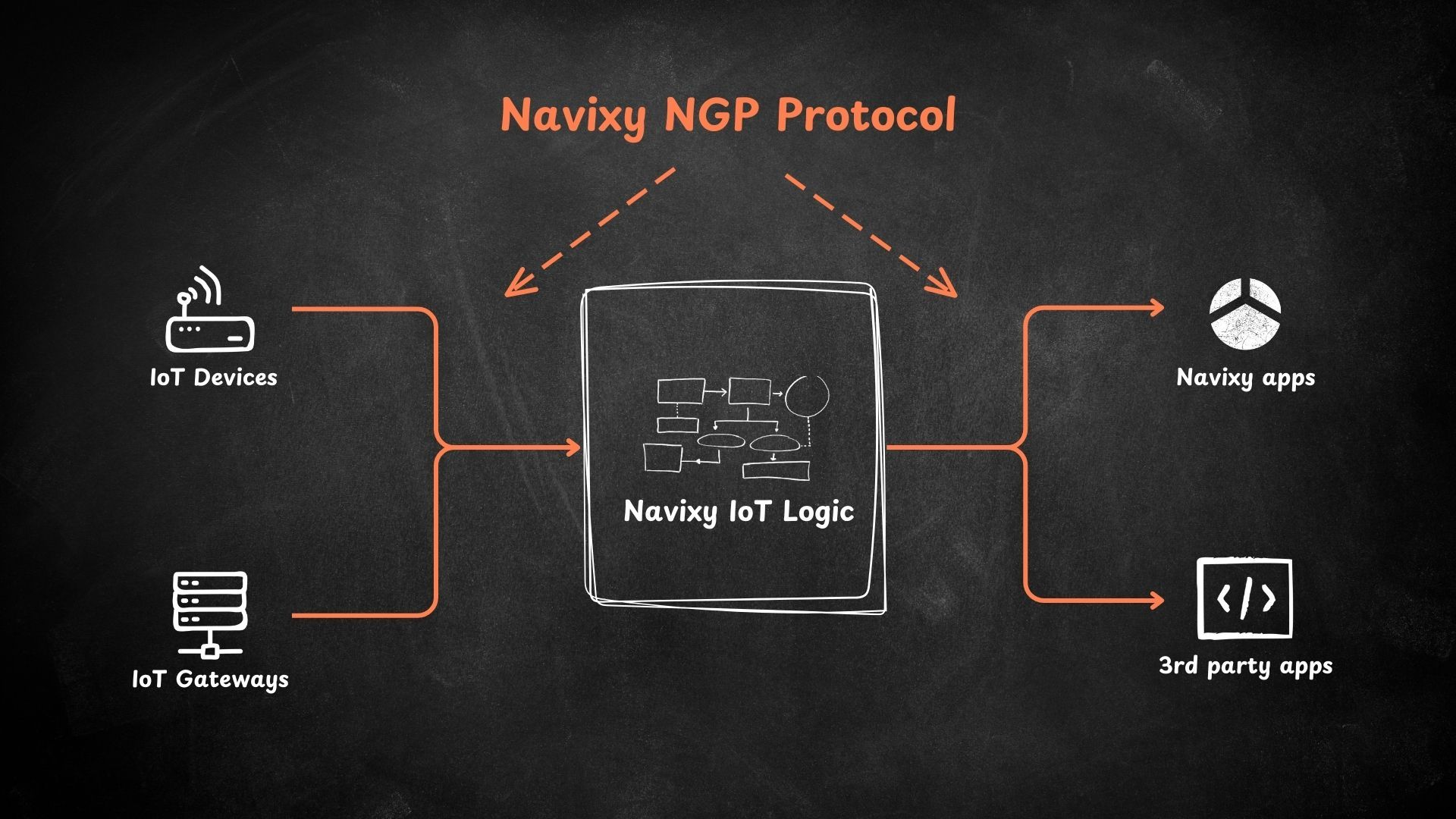
The upstream flow begins with data collection from the field. IoT devices and sensors transmit their readings—location coordinates, temperature measurements, engine diagnostics, and other telemetry—using NGP as their native protocol. For existing deployments, IoT gateways convert data from various proprietary protocols into NGP format, creating a unified data stream. This standardization extends to legacy systems through protocol adapters, preserving existing investments while modernizing the data pipeline.
This standardized data can be used directly by your applications, or it can undergo additional processing through systems like Navixy IoT Logic. When integrated with IoT Logic, raw device data can be enriched with business context, normalized across different sources, and validated for consistency. The processed data then flows downstream to various consumers—fleet management dashboards, maintenance scheduling systems, mobile apps, or third-party analytical platforms—using the same NGP format. This protocol's flexibility means you can adapt the output to meet specific integration requirements without compromising data integrity.
NGP in action: real-world solutions
Let's look at how different enterprises solved their integration challenges using NGP, each bringing unique requirements and complexity to the table.
Multi-vendor fleet modernization
A logistics company with over 5,000 vehicles faced a common enterprise challenge: their fleet used telematics devices from six different vendors, each with its own integration point and data format. After implementing NGP, they unified their entire telematics stack. Integration time for new devices dropped from weeks to days, and their development team could finally focus on building fleet optimization features instead of maintaining protocol parsers. The standardized data format also enabled their first company-wide fuel efficiency program, previously impossible due to inconsistent data across device types.
Cold chain compliance monitoring
A pharmaceutical distributor needed granular tracking of their temperature-controlled fleet while meeting strict compliance requirements. Their challenge intensified with the acquisition of a company using different tracking hardware. NGP gateways standardized the data from both legacy temperature sensors and modern telematics units. This unified approach streamlined their compliance reporting and enabled real-time temperature breach alerts across their entire fleet, regardless of the underlying hardware.
Heavy equipment fleet management
A construction company operating across 200 sites needed to track both owned and rented equipment from multiple manufacturers. Each OEM provided their own telematics system, creating a fragmented view of asset utilization. NGP unified the data streams from various equipment types—from excavators to portable generators—into a single format. This enabled them to build a comprehensive asset management system that tracks usage patterns, maintenance schedules, and fuel consumption across their entire fleet, dramatically improving equipment allocation and reducing idle time.
Business impact: how NGP transforms engineering
The adoption of NGP delivers distinct advantages for both telematics service providers and enterprise IT teams building internal fleet management solutions:
- For telematics service providers and system integrators, NGP delivers value at two critical points in the data pipeline. On the ingestion side, instead of maintaining separate protocols for each hardware vendor, you get a single, standardized way to collect device data. But the real power comes from NGP's application integration capabilities. Whether you're working with raw telemetry data or enriched data streams processed through components like IoT Logic, you can use the same protocol to feed this data into your various applications—from basic tracking interfaces to sophisticated analytics platforms. This unified approach means you can rapidly develop and deploy new applications without worrying about underlying data formats or building custom integration layers for each data source.
- Enterprise IT departments face similar challenges when building internal fleet management systems. NGP eliminates the need to juggle multiple vendor protocols and SDKs, simplifying both initial development and ongoing maintenance. Your team can build a single integration layer that handles all your current and future telematics devices. This standardization is particularly valuable when scaling operations—whether you're adding new vehicle types, expanding into new regions with different hardware suppliers, or integrating newly acquired fleets.
NGP's future-proof architecture and extensible design easily accommodates new device types and data formats, while backward compatibility ensures your existing integrations remain stable through protocol updates. This architectural stability, combined with reduced development and maintenance costs, delivers compelling ROI for any organization dealing with multi-vendor telematics solutions.
Under the hood: the technical foundation of NGP
Now that we’ve covered the business applications, let’s dive into the technical foundation of the Navixy Generic Protocol (NGP). The protocol is built on key design principles that enhance its flexibility, compatibility, and ease of use across diverse telematics applications. Here’s a breakdown of NGP’s core architecture:
JSON-first architecture |
|
Transport layer flexibility |
|
Extensible data model |
|
Forward compatibility |
|
While detailed protocol specifications are available in our documentation, we'll focus here on key design patterns and implementation approaches.
Core design patterns: making NGP work for you
Direct device integration
For hardware manufacturers looking to natively support NGP, the protocol offers a straightforward implementation path. The minimal viable integration requires just device identification and timestamp, with optional fields for extending functionality:
#include <stdio.h> #include <time.h> #include "ngp_client.h" typedef struct { double latitude; double longitude; float speed; uint8_t satellites; } gps_data_t; int send_telemetry(const char* device_id, const gps_data_t* gps_data) { char payload[256]; time_t now; time(&now); // Format minimal NGP message snprintf(payload, sizeof(payload), "{" "\"message_time\":\"%s\"," "\"device_id\":\"%s\"" "}", format_iso_time(now), device_id ); // Optionally add location data if GPS fix is valid if (gps_data && gps_data->satellites > 3) { char location[128]; snprintf(location, sizeof(location), ",\"location\":{" "\"latitude\":%.6f," "\"longitude\":%.6f," "\"speed\":%.1f," "\"satellites\":%d" "}", gps_data->latitude, gps_data->longitude, gps_data->speed, gps_data->satellites ); // Insert location before the closing brace insert_before_end(payload, location); } return mqtt_publish(payload); }
Protocol gateway pattern
For organizations with existing device fleets, NGP provides a gateway pattern that enables gradual migration:
class NGPGateway: def convert_to_ngp(self, legacy_data): # Start with required fields message = { "message_time": self._normalize_timestamp( legacy_data.timestamp ), "device_id": legacy_data.identifier } # Map device-specific fields if legacy_data.has_custom_fields(): message["custom_attributes"] = self._map_fields( legacy_data.fields ) return message
Event-driven updates
NGP supports both periodic updates and event-driven reporting. This pattern is particularly useful for optimizing data transmission:
class DeviceMonitor: def __init__(self, threshold=5.0): self.last_position = None self.movement_threshold = threshold def should_report(self, new_position): if not self.last_position: return True distance = calculate_distance( self.last_position, new_position ) return distance > self.movement_threshold
Implementation considerations
When implementing NGP, consider these key aspects:
- Transport selection
- Use MQTT for real-time tracking and bidirectional communication
- Choose HTTP for simple integrations or batch updates
- Consider HTTPS for enhanced security requirements
- Data optimization
- Send only changed values in periodic updates
- Use batch processing for historical data
- Implement appropriate QoS levels based on data criticality
- Error handling
- Implement retry logic for failed transmissions
- Cache data locally when connectivity is lost
- Validate data before transmission
These patterns provide a foundation for building robust NGP implementations. For detailed protocol specifications, message formats, and advanced features, refer to our protocol documentation. For technical questions and implementation support, reach out to our developer relations team.
What’s next for NGP
The telematics landscape is always shifting, and NGP is designed to keep pace. We’re focused on expanding its capabilities without sacrificing the simplicity and reliability it’s known for.
Our roadmap includes more transport options, like TCP and UDP, and support for Protocol Buffers (Protobuf) to improve data efficiency, along with optimizations for complex sensors and ML outputs. Security is a top priority too, with plans for enhanced encryption, authentication, and access control.
Our goal is to keep NGP at the forefront of telematics integration — making device connections simpler and faster for everyone. We’re also committed to community-driven growth, with open feedback channels and regular enhancement proposals to shape NGP’s future.
Wrapping up: NGP as a solution for telematics integration
The Navixy Generic Protocol is here to tackle one of telematics’ biggest headaches: integration. By offering a standardized, flexible, and well-documented protocol, we’re cutting down the technical roadblocks that hold back innovation in our industry.
Whether you’re a hardware manufacturer wanting to reach more users, a system integrator piecing together complex setups, or a telematics provider aiming to simplify device support, NGP has you covered with a practical, robust solution.
And we’re just getting started—this post kicks off our series on telematics standardization and integration. Stick around on our tech blog for more deep dives, case studies, and hands-on guides to keep you ahead of the curve.